How to easily run an HTTP server on macOS 14 Sonoma
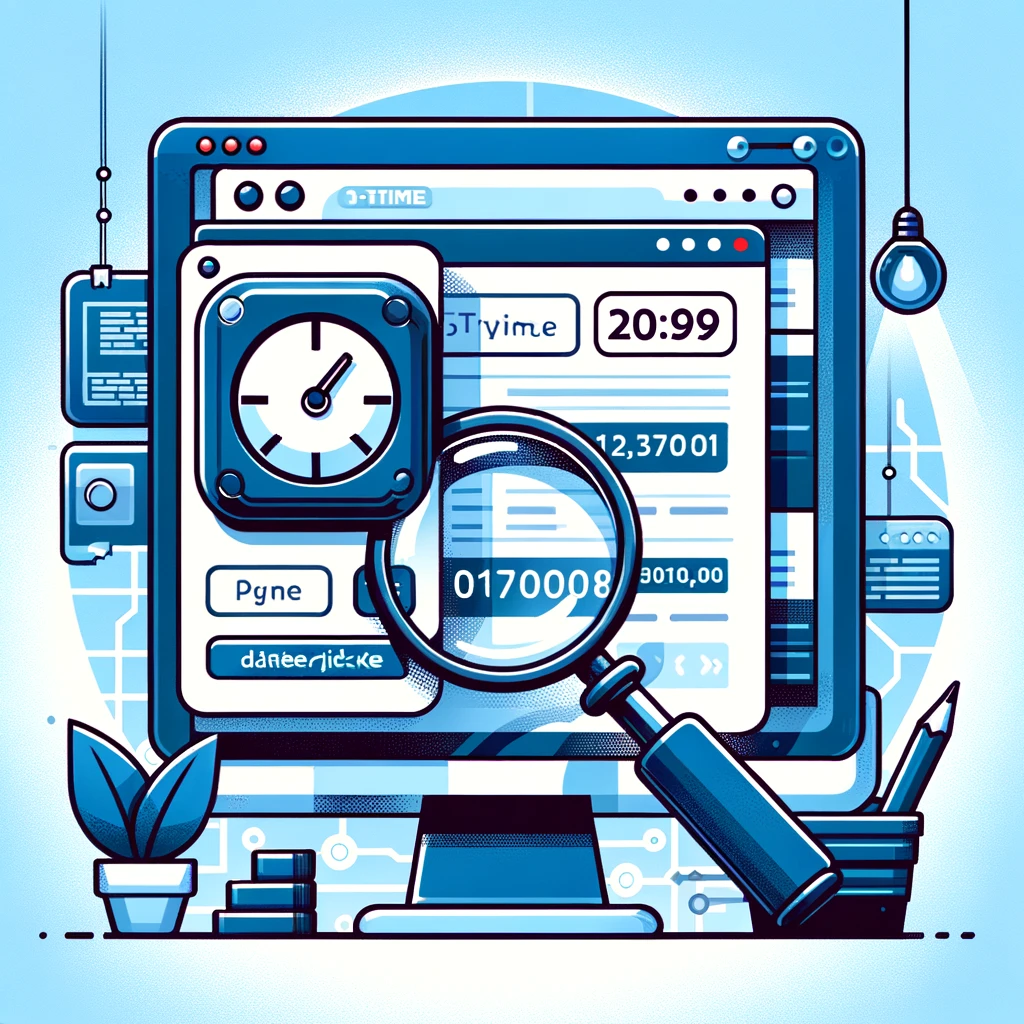
Running a local server is essential for web development, allowing you to preview your projects in a web browser. Python’s built-in HTTP server is a straightforward solution for this. In this article, I’ll guide you through setting up a convenient function in your .zshrc
file to run a Python HTTP server effortlessly on macOS 14 Sonoma.
Prerequisites
- macOS 14 Sonoma
- Basic knowledge of using the terminal
- Python 3 installed (we’ll cover installation if it’s not already set up)
What is an HTTP Server?
An HTTP server is a software application that serves content to clients, typically web browsers, over the HTTP protocol. It listens for incoming requests from clients and responds by delivering the requested resources, which can include HTML pages, images, CSS stylesheets, JavaScript files, and other types of content.
Python provides a straightforward and efficient way to run a simple HTTP server using its built-in http.server
module. This module can be used to serve files from a directory. To start a server using Python, you can use the following command:
python3 -m http.server 8000
Why You May Need It
As a web developer, running a local HTTP server is an essential part of your toolkit. Here are some reasons why you might need it:
- Testing Web Projects Locally: Before deploying your web projects to a live server, you can test them locally to ensure everything works as expected.
- Serving Static Files During Development: An HTTP server allows you to serve static files like HTML, CSS, and JavaScript, making it easier to develop and test your front-end code.
- Quickly Sharing Files Over a Local Network: You can use the server to quickly share files with other devices on the same network without the need for additional software.
The built-in HTTP server in Python is an excellent tool for web development and testing purposes.
The Challenge
While Python’s built-in HTTP server is convenient, using it frequently can present some challenges:
- Repetitive command entry: Manually typing the command to start the server can be repetitive and time-consuming, especially if you need to serve files from various directories or use different ports.
- Directory navigation disruption: To start the server you required to switch to the serving directory. Stopping the server leaves you in the served directory. This disruption often requires you to navigate back to your original working directory, impacting your workflow.
The Solution
To streamline the process of running a Python HTTP server, you can create a custom function in your .zshrc
file. This function will:
- Default to serving the current directory: If no directory is specified, the function will serve files from the current directory.
- Default to port 8000: If no port is specified, the function will use port 8000.
- Print a custom message: The function will print a message with the URL to navigate to in your web browser.
- Preserve the working directory: By running the server in a subshell, the function ensures that your working directory remains unchanged after stopping the server.
This approach simplifies the process of running an HTTP server, making it more efficient and less disruptive to your workflow.
Step-by-Step Guide
If Python 3 is not installed on your system, follow these steps:
- Check if Python 3 is installed:
python3 --version
- Install Homebrew (if not already installed):
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Homebrew is a package manager for macOS and Linux. The official website https://brew.sh
- Install Python 3 using Homebrew:
brew install python
Add the following function to your .zshrc
file to facilitate serving directories with Python’s HTTP server.
- Open your
.zshrc
file: Open your terminal and run:nano ~/.zshrc
- Add the
serve
function: Scroll to the bottom of the file and paste the following function:# HTTP server # Function to serve a directory using Python's built-in HTTP server # without changing the current working directory in the parent shell serve() { local dir=${1:-.} # Default to the current directory if no directory is provided local port=${2:-8000} # Default to port 8000 if no port is provided # Run the server in a subshell to avoid changing the parent shell's directory (cd "$dir" && echo "Navigate to http://localhost:$port in your web browser" && python3 -m http.server "$port" > /dev/null 2>&1) }
-
Save and close: Save the changes and close the editor (
CTRL + O
to save andCTRL + X
to exit). - Reload your
.zshrc
file: Apply the changes by reloading your.zshrc
file:source ~/.zshrc
You can now use the serve
command to start a Python HTTP server from any directory. Here are some examples:
Serve the current directory on port 8000:
serve
Serve a specific directory on port 8000:
serve path/to/directory
Serve a specific directory on a specific port:
serve path/to/directory 9000
When you run the serve
command, it will start the HTTP server and print a message indicating the URL to navigate to in your web browser. After stopping the server with CTRL+C
, your working directory will remain unchanged.
Conclusion
By adding this simple serve
function to your .zshrc
file, you can streamline the process of starting a Python HTTP server for your development projects on macOS 14 Sonoma. This not only saves time but also ensures your workflow remains smooth and efficient.
This guide has shown you how to overcome the repetitive nature of manually starting the server and the disruption caused by directory changes. By following these steps, you can now serve files from any directory without changing your current working directory, making your development process more seamless and productive.
I hope this guide has been helpful in improving your workflow with Python’s built-in HTTP server. If you have any questions or additional tips, feel free to leave a comment below Happy coding!
Thank you for taking the time to read this article. May your development environment remain efficient and hassle-free with your newly configured serve function!
Arthur is a designer and full stack software engineer. He is the founder of Space X-Chimp and the blog My Cyber Universe. His personal website can be found at arthurgareginyan.com.