Why is the code from my functions.php file not working from inside the plugin?
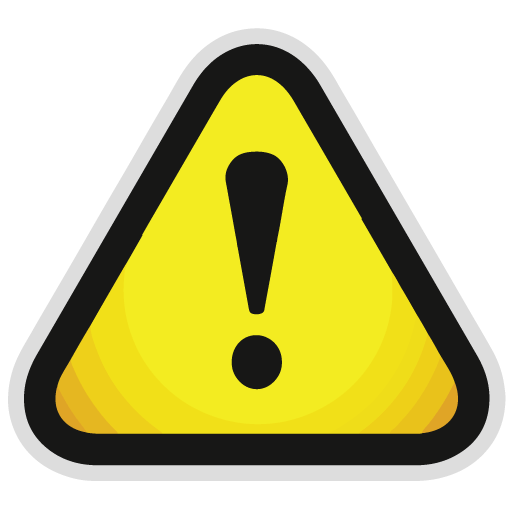
It happens that after moving a PHP code from the functions.php
file of the theme on a WordPress website to the functionality plugin, it stops working and even leads to an inaccessible of the website, although before that it worked. In this article I will explain why the custom PHP code does not work from inside the functionality plugin, and I show you how to solve this.
What causes this
This problem occurs due to the fact that the code inside the functions.php
file of the theme and the code inside the plugin are not triggered simultaneously, but at different moments.
Running PHP code at the wrong time is a crucial mistake that can break the entire website. Due to this mistake, you may get a white screen (WSOD / ERROR 500) and the following error message (an example):
Fatal error: Uncaught Error: Call to undefined function wc_gzd_get_hook_priority() in /Users/user/Sites/site/wp-content/plugins/my-custom-functions-pro/inc/php/functional.php(99) : eval()'d code:1 Stack trace: #0 /Users/user/Sites/site/wp-content/plugins/my-custom-functions-pro/inc/php/functional.php(99): eval() #1 /Users/user/Sites/site/wp-content/plugins/my-custom-functions-pro/inc/php/functional.php(110): spacexchimp_p011_exec() #2 /Users/user/Sites/site/wp-content/plugins/my-custom-functions-pro/my-custom-functions-pro.php(67): require_once('/Users/user/S...') #3 /Users/user/Sites/site/wp-settings.php(322): include_once('/Users/user/S...') #4 /Users/user/Sites/site/wp-config.php(82): require_once('/Users/user/S...') #5 /Users/user/Sites/site/wp-load.php(37): require_once('/Users/user/S...') #6 /Users/user/Sites/site/wp-admin/admin.php(31): require_once('/Users/user/S...') #7 /Users/user/Sites/site/wp-admin/options-general.php(10): require_once('/Users/user/S...' in /Users/user/Sites/site/wp-content/plugins/my-custom-functions-pro/inc/php/functional.php(99) : eval()'d code on line 1
In the example of the error message above, it is reported that the function wc_gzd_get_hook_priority()
is still undefined, so it cannot be used.
How to solve it
To solve this problem all you need to do is wrap your custom PHP code in an additional function that is called at the right time. For this, perform the following steps.
- Register a new function and place your custom PHP code inside this function.
function my_init_function() { // YOUR CODE HERE }
- Add the init hook with calling the function that we just created.
add_action( 'init', 'my_init_function');
The init hook fires after WordPress has finished loading but before any headers are sent. Most of WordPress is loaded at this stage, and the user is authenticated. WordPress continues to load on the init hook that follows (e.g. widgets), and many plugins instantiate themselves on it for all sorts of reasons (e.g. they need a user, a taxonomy, etc.). You can learn more about this hook at the following links: https://codex.wordpress.org/Plugin_API/Action_Reference/init https://codex.wordpress.org/Plugin_API/Action_Reference
Example
I know that an example is always clearer than just a numbered list of actions, so here’s an example.
Suppose that in the functions.php
file of the theme on our WordPress website, we have the following PHP code which we want to move to the functionality plugin:
// Add WooCommerce Germanized correct hooks position
add_action( 'astra_woo_shop_add_to_cart_before', 'woocommerce_gzd_template_single_price_unit', 8, wc_gzd_get_hook_priority( 'loop_price_unit' ) );
add_action( 'astra_woo_shop_add_to_cart_before', 'woocommerce_gzd_template_single_tax_info', 9, wc_gzd_get_hook_priority( 'loop_tax_info' ) );
add_action( 'astra_woo_shop_add_to_cart_before', 'woocommerce_gzd_template_single_delivery_time_info', 10, wc_gzd_get_hook_priority( 'loop_delivery_time_info' ) );
add_action( 'astra_woo_shop_add_to_cart_before', 'woocommerce_gzd_template_single_shipping_costs_info', 11, wc_gzd_get_hook_priority( 'loop_shipping_costs_info' ) );
// Uncheck WooCommerce delivery address true
add_filter( 'woocommerce_ship_to_different_address_checked', '__return_false' );
After we move the code above to the functionality plugin, the final code should look like this:
add_action( 'init', 'my_init_function');
function my_init_function() {
// Add WooCommerce Germanized correct hooks position
add_action( 'astra_woo_shop_add_to_cart_before', 'woocommerce_gzd_template_single_price_unit', 8, wc_gzd_get_hook_priority( 'loop_price_unit' ) );
add_action( 'astra_woo_shop_add_to_cart_before', 'woocommerce_gzd_template_single_tax_info', 9, wc_gzd_get_hook_priority( 'loop_tax_info' ) );
add_action( 'astra_woo_shop_add_to_cart_before', 'woocommerce_gzd_template_single_delivery_time_info', 10, wc_gzd_get_hook_priority( 'loop_delivery_time_info' ) );
add_action( 'astra_woo_shop_add_to_cart_before', 'woocommerce_gzd_template_single_shipping_costs_info', 11, wc_gzd_get_hook_priority( 'loop_shipping_costs_info' ) );
// Uncheck WooCommerce delivery address true
add_filter( 'woocommerce_ship_to_different_address_checked', '__return_false' );
}
Conclusion
That’s it, you’re done. Only three extra lines and now your custom PHP code that you move from the functions.php
file of the theme to the functionality plugin should work without errors. So simple isn’t it?
If you are having trouble fixing this problem with the instructions above, but are being able to solve this problem with any another method please describe it in the comment section below. Thanks!
If this article has helped you solve the problem then please leave a comment
Thanks for reading!
Arthur is a designer and full stack software engineer. He is the founder of Space X-Chimp and the blog My Cyber Universe. His personal website can be found at arthurgareginyan.com.